Mastering Fastify: Error Handling and Query String Configuration
Written on
Chapter 1: Introduction to Fastify
Fastify is a lightweight framework for Node.js designed for building backend web applications. In this guide, we will explore effective methods for implementing error handling and configuring query string options in Fastify.
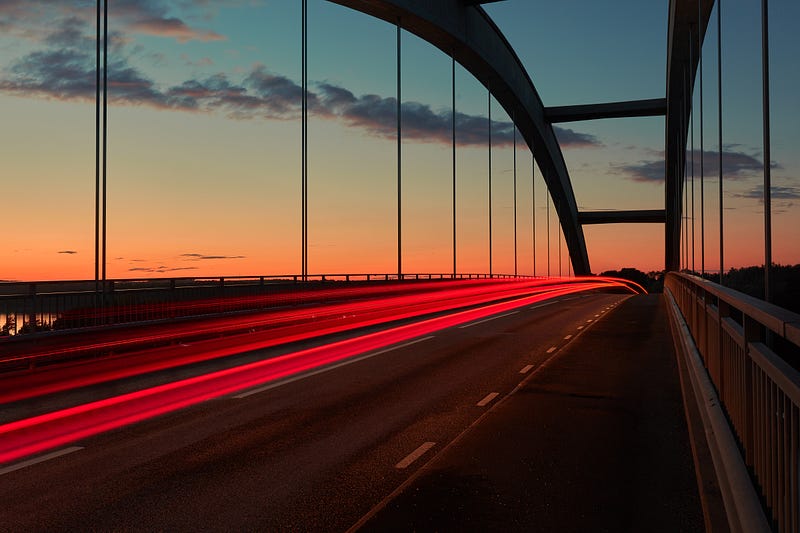
Chapter 2: Understanding Server Options
Fastify provides numerous configurable server options, including the trustProxy setting, which informs the framework that it operates behind a proxy server. This option can be set to either a specific proxy server's IP address or a boolean value. Alternatively, you can create a function to determine which proxy IPs should be trusted.
In your request object, you can access the following attributes: ip, ips, hostname, and protocol. Here’s a simple code example to demonstrate this:
let i = 0;
const fastify = require('fastify')({
genReqId(req) { return i++; }
});
fastify.get('/', (request, reply) => {
console.log(request.ip);
console.log(request.ips);
console.log(request.hostname);
console.log(request.protocol);
reply.send('hello world');
});
const start = async () => {
try {
await fastify.listen(3000, '0.0.0.0');} catch (err) {
fastify.log.error(err);
process.exit(1);
}
};
start();
Here, ip contains the client's IP address, hostname gives the host name, and protocol indicates the used protocol.
Section 2.1: Configuring Query String Parsing
The querystringParser option allows you to define how query strings are processed. For instance, you can utilize the qs library for parsing:
const qs = require('qs');
const fastify = require('fastify')({
querystringParser: str => qs.parse(str)
});
fastify.get('/', (request, reply) => {
reply.send('hello world');
});
const start = async () => {
try {
await fastify.listen(3000, '0.0.0.0');} catch (err) {
fastify.log.error(err);
process.exit(1);
}
};
start();
This setup employs the qs.parse method to convert query strings into an object.
Section 2.2: Versioning Routes
Fastify supports route versioning using semantic versioning (semver). You can implement versioning as follows:
const versioning = {
storage() {
let versions = {};
return {
get: (version) => { return versions[version] || null; },
set: (version, store) => { versions[version] = store; },
del: (version) => { delete versions[version]; },
empty: () => { versions = {}; }
};
},
deriveVersion: (req, ctx) => {
return req.headers['accept'];}
};
const fastify = require('fastify')({
versioning
});
fastify.get('/', (request, reply) => {
reply.send('hello world');
});
const start = async () => {
try {
await fastify.listen(3000, '0.0.0.0');} catch (err) {
fastify.log.error(err);
process.exit(1);
}
};
start();
In this example, methods like get, set, and del are utilized to manage the versions effectively.
Section 2.3: Error Handling in Fastify
Fastify allows the definition of custom error handlers for various types of errors encountered by the application. For example:
const fastify = require('fastify')({
frameworkErrors(error, req, res) {
if (error instanceof FST_ERR_BAD_URL) {
res.code(400);
return res.send("The provided URL is not valid");
} else {
res.send(err);}
}
});
fastify.get('/', (request, reply) => {
reply.send('hello world');
});
const start = async () => {
try {
await fastify.listen(3000, '0.0.0.0');} catch (err) {
fastify.log.error(err);
process.exit(1);
}
};
start();
Here, we define our own handler for bad URL errors, returning a 400 status code when necessary.
Section 2.4: Handling Client-Side Errors
The clientErrorHandler option allows you to respond to error events emitted by the client connection. You can configure it like this:
const fastify = require('fastify')({
clientErrorHandler(err, socket) {
const body = JSON.stringify({
error: {
message: 'Client error',
code: '400'
}
});
this.log.trace({ err }, 'client error');
socket.end(HTTP/1.1 400 Bad RequestrnContent-Length: ${body.length}rnContent-Type: application/jsonrnrn${body});
}
});
fastify.get('/', (request, reply) => {
reply.send('hello world');
});
const start = async () => {
try {
await fastify.listen(3000, '0.0.0.0');} catch (err) {
fastify.log.error(err);
process.exit(1);
}
};
start();
In this setup, we add our own clientErrorHandler to manage client-side errors effectively.
Chapter 3: Conclusion
In conclusion, Fastify offers a flexible framework that allows developers to implement various handlers to improve application performance and error management.
This video demonstrates error handling techniques using Fastify.
Learn how to build an authentication API with Node.js, TypeScript, and ExpressJS.